ANSI C language and libraries reference manual
October 1992
INMOS document number: 72-TDS-347-01
462 Pages
© INMOS Limited 1992.
About this manual
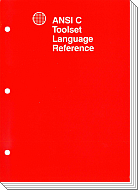
This manual is the Language and Libraries Reference Manual to the ANSI C toolset and provides a language reference for the toolset and implementation data.
The manual is divided into two parts: 'Runtime Library' and 'Language Reference', plus appendices.
The first section Runtime Library:
- Introduces the runtime library and summarizes the header files.
- Provides a detailed description of each library function, in alphabetical order.
- Describes how to modify the runtime startup system by removing segments not required by the user's application. Only very experienced users should attempt this.
The 'Language Reference' section describes:
- New features in the ANSI standard.
- ANSI C toolset language extensions.
- ANSI C toolset implementation details.
The three appendices cover:
- Syntax of language extensions.
- ANSI compliance data.
- Further explanation of the cyclic redundancy function provided.
Contents
Contents overview Contents Preface Host versions About this manual About the toolset documentation set Other documents occam and FORTRAN toolsets Documentation conventions
Runtime Library
1 Introduction and runtime library summary 1.1 Introduction 1.1.1 Accessing library functions 1.1.2 Linking libraries with programs 1.1.3 iserver protocols 1.1.4 Functions which store data in static 1.2 Header files 1.3 ANSI functions 1.3.1 Diagnostics <assert.h> 1.3.2 Character handling <ctype.h> 1.3.3 Error handling <errno.h> 1.3.4 Floating point constants <float.h> 1.3.5 Implementation limits <Iimits.h> 1.3.6 Localization <locale.h> 1.3.7 Mathematics library <math.h> 1.3.8 Non-local jumps <setjmp.h> 1.3.9 Signal handling <signal.h> 1.3.10 Variable arguments <stdarg.h> 1.3.11 Standard definitions <stddef.h> 1.3.12 Standard I/O <stdio.h> Characteristics of file handling 1.3.13 Reduced library I/O functions <stdiored.h> 1.3.14 General utilities <stdlib.h> 1.3.15 String handling <string.h> 1.3.16 Date and time <time.h> 1.4 Concurrency functions 1.4.1 Process control <process.h> 1.4.2 Channel communication <channel.h> 1.4.3 Semaphore handling <semaphor.h> 1.5 Other functions 1.5.1 I/O primitives <iocntrl.h> 1.5.2 float maths <mathf.h> 1.5.3 Host utilities <host.h> 1.5.4 Host channel access utilities <hostlink.h> 1.5.5 Boot link channel functions <bootlink.h> 1.5.6 MS-DOS system functions <dos.h> 1.5.7 Dynamic code loading functions <fnload.h> 1.5.8 Miscellaneous functions <misc.h> 1.6 Fatal runtime errors 1.6.1 Runtime error messages 2 Alphabetical list of functions 2.1 Format 2.1.1 Reduced library 2.1.2 Macros 2.2 List of functions 3 Modifying the runtime startup system 3.1 Introduction 3.2 Overview of system 3.3 The gsb and use of the IMS_nolink pragma 3.4 Interface to runtime startup code 3.5 Details of stage 1 of the runtime startup code 3.5.1 Initialize static 3.5.2 Call stage 2 startup code and set up gsb 3.6 Details of stage 2 of the runtime startup code 3.6.1 Set up bounds of stack 3.6.2 Initialize heap 3.6.3 Initialize pointer to configuration process structure 3.6.4 Initialize I/O system 3.6.5 Get command line arguments 3.6.6 Save exit return point 3.6.7 Initialize clock 3.6.8 Call main 3.6.9 Terminate server if required 3.7 Interface to main 3.8 Static initialization 3.9 Source files supplied and rebuilding UNIX based toolsets MS-DOS based toolsets VMS based toolsets 3.10 Notes 3.11 Example 3.11.1 Building the modified runtime system For example UNIX based toolsets MS-DOS/VMS based toolsets
Language Reference
4 New features in ANSI C 4.1 Summary of new features in the ANSI standard 4.2 Details of new features 4.2.1 Function declarations 4.2.2 Function prototypes 4.2.3 Functions without prototypes 4.2.4 Declarations 4.2.5 Types, type qualifiers and type specifiers 4.2.6 Constants 4.2.7 Preprocessor extensions Compiler directives Predefined macros 4.2.8 Structures and unions 4.2.9 Trigraphs Trigraph escape codes 5 Language extensions 5.1 Concurrency support 5.2 Pragmas 5.3 Predefined macros 5.4 Assembly language support 5.4.1 Directives and operations 5.4.2 size option on __asm statement 5.4.3 Labels 5.4.4 Notes on transputer code programming 5.4.5 Useful built-in variables 5.4.6 Transputer code examples Setting the transputer error flag Loading constants using literal operands Labels and jumps Jump tables Loading floating point registers Using align/word to return an element of a table Inserting raw machine code 6 Implementation details 6.1 Data type representation 6.1.1 Scalar types 6.1.2 Arrays 6.1.3 Structures Example 1 (structuring on a 32-bit processor) Example 2 (structuring on a 32-bit processor) 6.1.4 Unions 6.2 Type conversions 6.2.1 Integers 6.2.2 Floating point 6.3 Compiler diagnostics 6.4 Environment 6.4.1 Arguments to main Configured case Unconfigured case 6.4.2 Interactive devices 6.5 Identifiers 6.6 Source and execution character sets Shift states for encoding multibyte characters Integer character constants Locale used to convert multibyte characters Plain chars 6.7 Integer operations Bitwise operations on signed integers Sign of the remainder on integer division Right shifts on negative-valued signed integral types 6.8 Registers 6.9 Enumeration types 6.10 Bit fields 6.11 volatile qualifier 6.12 Declarators 6.13 Switch statement 6.14 Preprocessing directives Constants controlling conditional inclusion Date and time defaults 6.15 Static data layout 6.15.1 Local static data layout 6.15.2 Constant static objects 6.16 Calling conventions 6.16.1 Parameter Passing 6.16.2 Calling Sequence 6.16.3 Rules for aliasing between formal parameters 6.17 Runtime library
Appendices
A Syntax of language extensions A.1 Notation A.2 #pragma directive A.3 __asm statement B ANSI standard compliance data B.1 Translation B.2 Environment B.3 Identifiers B.4 Characters B.5 Integers B.6 Floating point B.7 Arrays and pointers B.8 Registers B.9 Structures, unions, enumerations, and bit fields B.10 Qualifiers B.11 Declarators B.12 Statements B.13 Preprocessing directives B.14 Library functions B.15 Locale-specific behavior C CRC Resume C.1 Summary of functions C.2 Cyclic redundancy polynomials C.2.1 Format of result C.3 Notes on the use of the CRC functions C.4 Example of use Index