Parallel FORTRAN User Guide
Version 2.1.3 - November 1990
578 Pages
© 3L Ltd
Introduction
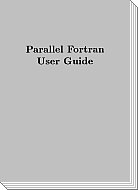
Intended Audience
This User Guide accompanies 3L's Parallel Fortran product, and is intended for anyone who wants to use Parallel Fortran to program a transputer system, whether writing a conventional sequential program or using the full support for concurrency which the transputer processor has to offer.
Hardware Assumptions
Parallel Fortran can be used with a large variety of target transputer systems. This manual makes the simplifying assumption that the target hardware will be an Inmos IMS B004 transputer evaluation board, or a transputer system which is largely compatible with a B004. This board is a single plug-in card for the standard IBM PC bus, with one transputer and either 1MB or 2MB of RAM.
Similarly, the assumption is made here that the host computer for the B004 will be an IBM PC with a hard disk drive, or one of the many personal computers compatible with the original IBM machines.
Document Structure
There are five main divisions within this document, as follows:
- Part I: Getting Started covers installing Parallel Fortran on your machine and verifying that it is operating correctly.
- Part II: Tutorial introduces you to the operation of the compiler and the other tools supplied with Parallel Fortran. In particular, there are tutorial sections explaining parallelism on the transputer and the way in which this can be accessed from Parallel Fortran programs.
- Part III: Language Reference contains a complete specification of the language accepted by the Parallel Fortran compiler. This is ANSI Fortran 77, with certain extensions which are described in this part.
- Part IV: General Reference contains the detailed technical information which you will require to write sophisticated applications for the transputer using Parallel Fortran.
- The appendices at the end of this manual contain supplementary information in a condensed form, such as tables of compiler error messages.
Further Reading
Although this User Guide does include a complete description of Fortran 77, readers who are unfamiliar with this language are advised to consult one of the many introductory texts available.
In a similar way, the reader is assumed to be reasonably familiar with the operating system of the host computer being used. For personal computers made by IBM, this will usually be PC-DOS, which is supplied with a manual called Disk Operating System Reference[2].
For compatible machines made by other manufacturers, the operating system will usually be MS-DOS, described in Microsoft MS-DOS User's Reference[3]. These two operating systems are largely compatible, and their documentation is very similar. We will refer to "MS-DOS" in this manual to mean the operating system used on your machine. The term DOS Reference Manual will be used to refer to the appropriate manual.
References to these and other documents mentioned in this manual are collected in a bibliography, which can be found on page 541.
Conventions
Throughout this manual, text printed in this typeface represents direct verbatim communication with the computer: for example, pieces of Fortran text, commands to MS-DOS and responses from the computer.
In examples, text printed in this typeface is not to be used verbatim: it represents a class of items, one of which should be used. For example, this is the format of the Fortran ASSIGN statement:
ASSIGN label TO int
This means that the statement consists of:
- The word 'ASSIGN', typed exactly like that.
- A label: not the word 'label', but something which the accompanying description explains.
- The word 'TO', typed exactly like that.
- An int: once again, the accompanying description explains what this is.
In examples, it is sometimes necessary to indicate exactly where there is a space, or how many spaces are present. In these cases, we represent a space by the symbol ''.
Contents
Introduction Intended Audience Hardware Assumptions Document Structure Further Reading Conventions
I Getting Started
1 Installing the Compiler 2 Confidence Testing
II Tutorial
3 Developing Sequential Programs 3.1 Editing 3.2 Compiling 3.3 Linking 3.3.1 Linking More than One Object File 3.3.2 Indirect Files 3.3.3 Calling the Linker Directly 3.3.1 Libraries 3.4 Running 3.4.1 Using Fortran Programs as MS-DOS Commands 3.4.2 I/O Units, Redirection and Piping 3.5 Memory Use 3.5.1 Default Memory Mapping 3.5.2 Alternative Memory Mapping 3.5.3 Limit on Program Memory 3.6 Accessing MS-DOS Functions 4 Introduction to Parallel Fortran 4.1 Abstract Model 4.2 Hardware Realisation 4.3 Software Model 4.4 Multiple Input Channels 4.5 Parallel Execution Threads 4.6 Configuring an Application 4.7 Processor Farms 5 Developing Parallel Programs 5.1 Configuring One User Task 5.1.1 Hardware Configuration 5.1.2 Software Configuration 5.1.3 Building the Application 5.2 More than One User Task 5.2.1 Inter-Task Communication Functions 5.3 Building Multi-Task Systems 5.4 Multi-Transputer Systems 5.5 Multi-Channel Input 5.5.1 The ALT Functions 5.6 Multi-Threaded Tasks 5.6.1 Threads versus Tasks 5.7 Debugging 5.8 Estimating Memory Requirements 6 Global Input/Output 6.1 One Transputer 6.2 More than One Transputer 6.3 More than One Multiplexer 6.4 Limits 6.5 Termination of an Application 7 Processor Farms 7.1 The Worker Task 7.2 The Master Task 7.3 The NET Package 7.3.1 F77_NET_SEND and F77_NET_RECEIVE 7.3.2 F77_NET_BROADCAST 7.4 Building the Application 7.4.1 Configuration File 7.5 Running the Example 7.6 Heterogeneous Networks
III Language Reference
Introduction 8 Fundamentals 8.1 Character Set 8.2 Program Structure 8.3 Program Unit Structure 8.3.1 Lines 8.3.2 Statements 8.3.3 Statement Labels 8.3.4 Categories of Statement 8.3.5 Order of Statements and Lines 8.4 Names 9 Data 9.1 Data Values and Types 9.2 Constants, Variables, and Arrays 9.2.1 Constants 9.2.2 Symbolic Constants 9.2.3 Variables 9.2.4 Arrays 9.2.5 Character Substrings 9.3 Type Specification 9.3.1 Predefined Specification 9.3.2 The IMPLICIT Statement 9.3.3 The IMPLICIT NONE Statement 9.3.4 The IMPLICIT UNDEFINED Statement 9.3.5 Explicit Type Specification Statements 9.3.6 The PARAMETER Statement 10 Storage of Data 10.1 Storage Requirements 10.1.1 Constants and Variables 10.1.2 Arrays 10.1.3 Character Storage 10.2 Allocation of Storage 10.2.1 General Considerations 10.2.2 The DIMENSION Statement 10.2.3 The COMMON Statement 10.2.4 The EQUIVALENCE Statement 10.3 Assignment of Initial Values 10.3.1 The DATA Statement 10.3.2 Block Data Subprogram 11 Expressions 11.1 Arithmetic Expressions 11.1.1 Arithmetic Elements 11.1.2 Arithmetic Operators and Parentheses 11.1.3 Rules 11.1.4 Order of Evaluation 11.1.5 Examples of Arithmetic Expressions 11.1.6 Determination of the Type of an Expression 11.1.7 Integer Arithmetic 11.1.8 Arithmetic Constant Expressions 11.1.9 Integer Constant Expressions 11.1.10 Not-a-Number and Infinity 11.2 Character Expressions 11.2.1 Character Elements 11.2.2 Character Operator and Parentheses 11.3 Logical Expressions 11.3.1 Logical Elements 11.3.2 Relational Expressions 11.3.3 Logical Operators and Parentheses 11.3.4 Rules 11.3.5 Order of Evaluation 11.3.6 Examples of Relational and Logical Expressions 12 Assignment Statements 12.1 Arithmetic Assignment Statements 12.2 Logical Assignment Statements 12.3 Character Assignment Statements 13 Control Statements 13.1 GO TO Statements 13.1.1 Unconditional GO TO 13.1.2 Computed GO TO 13.1.3 Assigned GO TO and ASSIGN Statements 13.2 IF Statements 13.2.1 Arithmetic IF 13.2.2 Logical IF 13.2.3 Block IF 13.3 DO Loops 13.3.1 DO Statements 13.3.2 The DO WHILE Statement 13.3.3 Terminal Statements 13.3.4 Nested DO-Loops 13.3.5 Transfer of Control in DO-Loops 13.4 The CONTINUE Statement 13.5 STOP Statements 13.6 PAUSE Statements 14 Program Units and the Transfer of Control 14.1 Procedures 14.1.1 Differences between Function and Subroutine Subprograms 14.1.2 Functions 14.1.3 Subroutines 14.2 Transfer of Control between Program Units 14.2.1 Functions 14.2.2 Subroutines 14.3 Correspondence between Dummy and Actual Arguments 14.3.1 Use of Constants and Expressions 14.3.2 Use of Variables 14.3.3 Use of Arrays and Array Elements 14.3.4 Use of Functions and Subroutines as Arguments 14.3.5 INTRINSIC Statement 14.4 Transfer of Values between Program Units 14.4.1 Common block items 14.4.2 Dummy and Actual Arguments 14.5 Multiple Entry into a Subprogram 14.5.1 The ENTRY Statement 14.5.2 Referencing an ENTRY Statement 14.5.3 Entering the Subprogram 14.5.4 Exit from the Subprogram 14.6 The SAVE Statement 14.7 The INCLUDE Statement 15 Format Specification 15.1 Format Specifications 15.1.1 Field Separators 15.1.2 Slash Editing 15.1.3 Repetition of Descriptors 15.2 Format Specification Methods 15.2.1 The FORMAT Statement 15.2.2 Character Format Specification 15.2.3 Effect of FORMAT Statements and Character Format Specifications 15.3 Edit Descriptors 15.3.1 Format (Conversion) Codes 15.3.2 Colon Editing 15.3.3 Default Field Widths 15.4 Examples of Format Specification 16 Input and Output 16.1 Introduction 16.1.1 Format of Records 16.1.2 Accessing Records 16.2 Input/Output Statements 16.2.1 Input/Output Lists 16.2.2 Correspondence Between Input/Output Lists and Format Codes 16.2.3 Implied DO-Loops 16.3 Sequential Access Input and Output 16.3.1 READ and WRITE Statements 16.3.2 File Positioning Input/Output Statements 16.4 Direct Access Input and Output 16.4.1 READ and WRITE statements 16.4.2 Formatted Direct Access Input and Output 16.4.3 Unformatted Direct Access Input and Output 16.5 List-Directed Input and Output 16.5.1 The READ Statement 16.5.2 Input Data 16.5.3 Output Statements 16.5.4 Output Data 16.6 Namelist-Directed Input and Output 16.6.1 The NAMELIST statement 16.6.2 Input Statements 16.6.3 Input Data 16.6.4 Output Statements 16.6.5 Output Data 16.6.6 Example of Namelist-Directed I/O 16.7 Internal Files 16.8 Auxiliary Input/Output Statements 16.8.1 Unit and File Connection 16.8.2 The OPEN Statement 16.8.3 The CLOSE Statement 16.8.4 The INQUIRE Statement
IV General Reference
17 Fortran Compiler Reference 17.1 Running the Compiler 17.2 Compiler Switches 17.2.1 Default switches 17.2.2 Controlling Source Processing 17.2.3 Controlling Output Files 17.2.4 Controlling Object Code 17.2.5 Controlling Debugging 17.2.6 Controlling INCLUDE Processing 17.2.7 Controlling the Format of the Listing 17.2.8 Information from the Compiler 17.2.9 Controlling the Compiler's Buffer Sizes 17.2.10 Obsolescent Switches 17.3 Handling of INCLUDE Files 17.4 Data-Type Representations 17.5 Data File Formats 17.5.1 FORMATTED SEQUENTIAL 17.5.2 FORMATTED DIRECT 17.5.3 UNFORMATTED SEQUENTIAL 17.5.4 UNFORMATTED DIRECT 17.6 Fortran Error Messages 17.6.1 Syntax Errors 17.6.2 Code Generator Errors 17.6.3 Fatal Errors 17.6.4 Run-Time Errors 18 The Parallel Fortran Run-Time Library 18.1 Purpose of the Run-Time Library 18.2 Non-Intrinsic Subprograms 18.2.1 Conventions 18.2.2 The DOS Package 18.2.3 The THREAD Package 18.2.4 The SEMA Package 18.2.5 The TIMER Package 18.2.6 The CHAN Package 18.2.7 The NET Package 18.2.8 The ALT Package 18.2.9 Compatibility Subroutines 18.2.10 Miscellaneous 19 The Linker 19.1 Command Line 19.2 File Name Conventions 19.3 The Output File 19.4 Indirect Files 19.5 Libraries 19.6 The Executable Image 19.7 Map Files 19.8 Debug Tables 19.9 Summary of Switches 19.10 Using Batch Files 19.11 Duplicate Definitions 19.12 Messages 20 The mempatch Utility 20.1 Identifying mempatch 20.2 Invoking mempatch 20.3 Re-invoking mempatch 21 The decode Utility 21.1 Usage 21.2 Features of the decode Program 21.3 Other Languages 22 The Worm Utility 22.1 Notes 23 The trim Utility 24 The tunlib Utility 25 The fpr Utility 26 Configuration Language Reference 26.1 Standard Syntactic Metalanguage 26.2 Configuration Language Syntax 26.2.1 Low Level Syntax 26.2.2 Numeric Constants 26.2.3 String Constants 26.2.4 Identifiers 26.2.5 Statements 26.2.6 PROCESSOR Statement 26.2.7 WIRE Statement 26.2.8 TASK Statement 26.2.9 CONNECT Statement 26.2.10 PLACE Statement 26.2.11 BIND Statement 27 Flood-Fill Configurer Reference 27.1 User Task Protocol 27.1.1 Master Task's Ports 27.1.2 Worker Task's Ports 27.2 Packet Format 28 Task Data Sheets
Appendices
A Distribution Kit A.1 Directory \tf2v1 A.2 Directory \tf2v1\examples B Compatibility with T414A and T800A B.1 Problems with T414A B.1.1 Restriction on Message Lengths B.1.2 Problems with Timers B.2 Problems with T800A B.2.1 Floating-Point Conversion Problems B.2.2 Instruction Decode Problems C Building a Network C.1 Network Principles C.2 Network Requirements C.2.1 Requirements for Links C.2.2 Requirements for System Services C.3 Connecting a Network D Additional Language Features D.1 The ENCODE and DECODE statements D.2 The DEFINE FILE statement D.3 Record selection D.4 The FIND Statement E Intrinsic Functions E.1 ANSI Standard Intrinsic Functions E.1.1 Rounding E.1.2 Character Type Conversion E.1.3 Numeric Type Conversion E.1.4 Arithmetic E.1.5 Maximum and Minimum E.1.6 Complex Operations E.1.7 Exponential and Logarithms E.1.8 Trigonometrical Functions E.1.9 Trigonometrical Functions (Degree) E.1.10 Hyperbolic Functions E.1.11 Character Operations E.1.12 Lexical Character Comparisons E.2 Bit-Manipulation Functions E.2.1 Bitwise Logical Operations E.2.2 Single-Bit Functions E.2.3 Shift and Extract F Summary of Option Switches F.1 Compiler Switches F.2 Linker Switches F.3 afserver Switches G Syntax Error Messages H Linker Error Messages I Run-Time Error Messages I.1 General Input/Output Errors I.2 Run-Time Format Errors I.3 Errors Returned by afserver J Mandelbrot Program Listings J.1 Master Task J.2 Worker Task J.3 Command Packet Include File J.4 Results Packet Include File J.5 Flood Configuration File J.6 Static Configuration File K ASCII Code Chart Bibliography Index