T9000 Transputer Instruction Set Manual
First Edition 1993
INMOS document number: 72-TRN-240-01
490 Pages
© INMOS Limited 1993. INMOS reserves the right to make changes in specifications at any time and without notice. The information furnished by INMOS in this publication is believed to be accurate; however, no responsibility is assumed for its use, nor for any infringement of patents or other rights of third parties resulting from its use. No license is granted under any patents, trademarks or other rights of INMOS.
Introduction
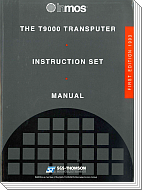
This book describes each instruction in the IMS T9000 instruction set and explains the context within which that instruction is used. It is essentially divided into two parts: a narrative that introduces each instruction within a logical group of instructions, and a reference section that gives a code-like specification of each instruction with a cross reference to the narrative.
[For details of the T9000 products and development tools, refer to The T9000 Hardware Reference Manual.]
This is a useful document to all transputer users, but it is aimed in particular at the following.
- the high-level programmer who wants to use low-level code inserts to enhance the performance of his code
- the compiler writer
- the operating system or run-time kernel writer
- the writer of high-level debugging tools
- the writer of run-time support libraries
- the writer of bootstrap code
The high-level programmer in a language such as C might find that, either his particular compiler is limited in certain respects, or a particular piece of code is time critical, and may hence need to write a low-level code sequence. Provided that the compiler/linker system that he is using enables him to write instruction level sequences into his program, he can overcome such difficulties. For this though he needs a good understanding of the capabilities and range of the entire instruction set.
A compiler writer needs to understand the exact action of each instruction in order to write the code generation part of his program.
The writer of a run-time kernel needs to understand the transputer's scheduling mechanism and the instructions which enable him to implement his own scheduling/interrupt scheme.
Similarly the writer of run time support libraries may need to write time critical code at assembly level.
The writer of a debugging tool needs to be able to control low-level context switching (e.g. for implementing breakpoints/single-step) and needs to be able to access and manipulate certain registers and data structures.
Bootstrap code must usually be very compact code and requires some machine specific instructions which are not available in high-level programming languages. For these reasons, a bootstrap program is written at assembly code level and the writer of the program needs to be familiar with the processor's instruction set.
In the narrative part of the book, the art of programming the IMS T9000 is considered subject by subject. Each subject introduces certain instructions. This provides the reader with the purpose for the instructions as they are introduced and describes any environmental issues, such as data structures and register/datastructure pre-conditions. Some of these subjects are of interest to all IMS T9000 users while some are of specific interest to certain categories of reader.
Chapters 3 to 8 should be read by all transputer programmers who are likely to need to know anything about the instruction set. The basic concepts of the IMS T9000 are introduced, including: addressing, instruction representation, processes, registers, communication, and the instructions which are essential for sequential and concurrent programming. These chapters may also be of interest to high-level programmers or system designers who would like a general background on how the IMS T9000 works.
Chapters 9 and 10 are concerned with running code under protection, implementing a memory management scheme, and handling errors or unexpected behaviour. They are of primary interest to operating system writers.
Chapter 11 describes the support for floating-point arithmetic and is of interest to compiler writers, for implementing mathematical run-time library support, and to programmers who are required to write IEEE floating-point exception-handlers.
Chapter 12 discusses how channels are used to communicate between transputer processes. In particular, it describes virtual channels, event channels and resource channels. This information is needed if writing a program to configure a network of transputers (a configurer).
Chapter 13 overviews the various context switches and associated state storage and retrieval mechanisms. These include traps, high-priority process interruption (and return), descheduling and timeslicing. Instructions are described which enable process queues to be manipulated and interrupts/timeslices to be enabled/disabled.
Chapter 14 discusses the various mechanisms available for monitoring the behaviour of a process as it runs, including: breakpointing, watchpointing, single-stepping. The instructions and mechanisms here are of interest to the programmer implementing debugging tools.
Chapter 15 provides a brief overview of the memory architecture and describes the instructions that can be used to invalidate and flush the main cache.
Appendix A is the instruction reference section. It lists all the IMS T9000 instructions in alphabetical order. For each instruction, there is a short English description, a pseudo-code description, and list of pre-conditions, a list of any conditions which may be set by the instruction, and cross reference to the page(s) in the narrative section of the book where the instruction is introduced.
Appendix B provides a tabulated list of all the instructions in ascending order of operation codes. This list is useful for disassembly of instruction code. That is, it provides the user with the information needed to convert from a hex code sequence to an instruction code sequence.
Contents
List of tables 1 Introduction 2 Notation, conventions and terminology 3 An overview 3.1 Processes 3.1.1 The occam process model 3.1.2 Implementation of processes 3.2 Communication 3.3 Traps 3.4 Configuration of control system 3.5 Instructions and pipelining 4 Addressing and data representation 4.1 Word address and byte selector 4.2 Ordering of information 4.3 Words, objects and signed integers 4.4 Unaligned address detection 5 Registers, status bits and control bits 5.1 Machine registers 5.1.1 State registers 5.1.2 Other machine registers 5.2 Process status and control bits 5.3 The process descriptor and its associated register fields 6 Instruction representation 6.1 Instruction encoding 6.1.1 An instruction component 6.1.2 The instruction data value and prefixing 6.1.3 Primary Instructions 6.1.4 Secondary instructions 6.1.5 Summary of encoding 6.2 Generating prefix sequences 6.2.1 Prefixing a constant 6.2.2 Evaluating minimal symbol offsets 7 Sequential operations 7.1 Registers 7.2 Local variables and constants, and stack operations 7.3 Integer stack evaluation 7.3.1 Loading operands 7.3.2 Tables of constants 7.3.3 Single length signed integer arithmetic 7.3.4 Single length modulo integer arithmetic 7.3.5 Unary minus 7.3.6 Fractional arithmetic 7.3.7 Bitwise logic and shifts 7.4 Non-local variables 7.5 Arrays and subscripts 7.5.1 Counting bytes and words 7.5.2 Forming addresses 7.5.3 Arrays 7.5.4 Transferring array elements 7.6 Multiple assignment 7.7 Comparisons and jumps 7.7.1 Representation of true and false 7.7.2 Comparisons 7.7.3 Implementation of languages with different representations of true and false 7.7.4 Boolean negation 7.7.5 Jump and conditional jump 7.7.6 Evaluation of boolean expressions 7.7.7 Conditional transfer of control 7.7.8 Compiling CASE statements 7.8 Long arithmetic and shifts 7.8.1 Multiple length addition and subtraction 7.8.2 Multiple length multiplication and division 7.8.3 Multiple length shifts 7.8.4 Normalizing 7.9 Object length conversion 7.9.1 Conversion between 8/16-bit object and word representations 7.9.2 Conversion between single word and double word representations 7.9.3 General conversion between N-bit object and word representations 7.10 Replication 7.11 Procedures 7.11.1 Adjusting workspace 7.11.2 Call and return 7.11.3 Use of (Wptr+0) 7.11.4 Loading parameters 7.11.5 The static chain 7.11.6 Other calling techniques 7.11.7 Other workspace allocation techniques 7.12 Functions 7.12.1 Calling a function 7.12.2 Single result functions 7.13 Error checking instructions 7.14 Device access instructions 7.15 Specialist instructions 7.15.1 Two dimensional block move 7.15.2 Bit manipulation and CRC evaluation 8 Concurrent processes 8.1 Workspace 8.1.1 Process workspace data structure 8.1.2 Size of workspace 8.2 Scheduling and priority 8.2.1 The current process, the null process, and scheduling lists 8.2.2 Descheduling 8.2.3 Rescheduling after communication 8.2.4 Clocks and timeslicing 8.2.5 Priorities and interruption 8.2.6 Scheduling/descheduling of L-processes 8.3 Initiation and termination of processes 8.3.1 Scheduling parallel processes 8.3.2 Other scheduling instructions 8.4 Channel communication, synchronization and data-transfer 8.4.1 Channels 8.4.2 Synchronization 8.4.3 Communication 8.4.4 Implementation of channels 8.5 Time 8.5.1 Past and future 8.5.2 Reading the clock 8.5.3 Timer input 8.5.4 Timer lists 8.6 Semaphores 8.7 Alternative input 8.7.1 The occam ALT construct 8.7.2 The 'alternative sequence' 8.7.3 Execution of the alternative sequence 8.7.4 Compiling an ALT statement 8.7.5 Trapping degenerate alternatives 8.7.6 Replicated ALT 8.7.7 PRI ALT 8.8 Resource channels 8.8.1 The client-server model 8.8.2 Resource mechanism and data structures 9 Protection and memory management 9.1 The mechanism 9.2 Instruction protection - privileged instructions 9.3 Address translation, memory protection, and stack extension 9.4 Regions 9.5 Region descriptors 9.6 Registers 9.7 Data structures 9.7.1 P-state data structure (PDS) 9.7.2 Region descriptor data structure (RDDS) 9.8 Instructions 10 The trap mechanism 10.1 The trap-handler 10.1.1 The THDS (trap-handler data structure) 10.1.2 Sharing a trap-handler data structure 10.1.3 The null trap-handler 10.2 State storage and retrieval when a trap is taken 10.3 Trap causes and signalling of errors 10.3.1 Trap causes 10.3.2 Signalling of errors 10.3.3 Null trap causes 10.4 Instructions 11 Floating-point instructions 11.1 IEEE floating-point arithmetic 11.2 The implementation of IEEE floating-point arithmetic on the IMS T9000 11.2.1 Formats 11.2.2 Floating-point operations 11.2.3 Exceptions 11.2.4 Not-a-Number representations (NaNs) 11.2.5 Implementation of underflow 11.3 Floating-point stack 11.4 Loading and storing floating-point values 11.4.1 Loading 11.4.2 Storing 11.5 Compiling floating-point expressions 11.6 Floating-point rounding mode 11.7 Floating-point arithmetic instructions 11.7.1 Dyadic operations 11.7.2 Monadic operations 11.8 Remainder and range instructions 11.9 Comparisons 11.9.1 Comparison instructions 11.9.2 Implementation of IEEE comparisons 11.9.3 Some anomalies 11.10 Class analysis 11.11 Type conversion 11.11.1 REAL to REAL conversions 11.11.2 REAL to INT conversions 11.11.3 INT to REAL conversions 11.12 Floating-point state 11.12.1 Floating-point status word 11.12.2 Saving the floating-point state 11.12.3 Instructions for saving and loading floating-point state 11.13 Exception handling mechanism 11.13.1 State delivered by floating-point exception - Implementing an IEEE (trap) handler 11.13.2 Some anomalies 11.14 Implementation of NaNs 12 Channels 12.1 Compilation and configuration of channels - an overview 12.2 External channels 12.2.1 Virtual channels 12.2.2 Byte-stream channels 12.2.3 Event channels 12.3 Channel states and modes of operation 12.3.1 Normal channel states 12.3.2 Resource channel states 12.3.3 Virtual and event channel activation modes 12.4 Channel configuration and mapping 12.4.1 Configuration register instructions 12.4.2 Configuration registers used for memory mapping 12.4.3 Virtual link mapping functions 12.4.4 Packet header labelling 12.5 Other configuration registers for setting up links and VCP 12.6 Setting up the virtual link control blocks 12.6.1 Instructions for setting up a VLCB 12.6.2 Null header 12.6.3 An example 12.7 Resource channels 12.7.1 Implementation of internal resource channels 12.7.2 Implementation of external resource channels 12.7.3 Reverse channel 12.7.4 Instructions for setting and using the resource mechanism 12.7.5 Usage of resource channels 12.8 Resetting and stopping a channel 12.8.1 Dealing with a communication failure 12.8.2 Recovering the use of a virtual channel which is in operation 12.9 Channel instructions according to usage 12.9.1 Instructions that can be applied to all channels 12.9.2 Instructions that can be applied to resource channels 12.9.3 Instructions that can be applied to external channels 12.9.4 Instructions that can be applied to virtual channels 13 Process state 13.1 Context switching 13.2 Partial context switch - descheduling and trapping 13.2.1 Descheduling and execution of the next process 13.2.2 Trapping 13.2.3 Instructions that are used to store and retrieve additional state 13.3 Full context switch - interruption 13.4 Restarting an interrupted process 13.5 Enabling and disabling interruption and timeslicing, and forcing a timeslice 13.6 Scheduling list and timer list queue manipulation 14 Debugging mechanisms 14.1 Breakpoints 14.2 Single-stepping 14.2.1 Single-stepping a P-process 14.2.2 Single-stepping an L-process 14.2.3 Early 'single-step' trap 14.3 Watchpoints 14.3.1 Watchpointing a P-process 14.3.2 Watchpointing an L-process 14.4 Single-stepping and watchpointing an L-process - some special considerations 15 Cache instructions 15.1 Workspace cache 15.2 Main Cache 15.3 Instructions A T9000 instruction set reference guide A.1 Introduction A.1.1 Instruction name A.1.2 Code A.1.3 Description A.1.4 Definition A.1.5 Error signals A.1.6 Comments A.2 Notation A.2.1 The transputer state A.2.2 General A.2.3 Undefined values A.2.4 Data types A.2.5 Representing memory A.2.6 The configuration subsystem A.2.7 Constants A.2.8 Operators A.2.9 Functions A.2.10 Conditions to instructions A.3 Instruction set definition A adc n add ajw n alt altend altwt and B bcnt bitcnt bitrevnbits bitrevword bsub C call n causeerror cb cbu ccnt1 chantype cir ciru cj n crcbyte crcword cs csngl csu csub0 cword D devlb devls devlw devmove devsb devss devsw diff disc disg diss dist div dup E enbc enbg enbs enbt endp eqc n erdsq F fdca fdcl fmul fpabs fpadd fpadddbsn fpb32tor64 fpchki32 fpchki64 fpdiv fpdivby2 fpdup fpeq fpexpdec32 fpexpinc32 fpge fpgt fpi32tor32 fpi32tor64 fpint fpldall fpldnladddb fpldnladdsn fpldnldb fpldnldbi fpldnlmuldb fpldnlmulsn fpldnlsn fpldnlsni fpldzerodb fpldzerosn fplg fpmul fpmulby2 fpnan fpnotfinite fpordered fpr32tor64 fpr64tor32 fprange fprem fprev fprm fprn fprp fprtoi32 fprz fpsqrt fpstall fpstnldb fpstnli32 fpstnlsn fpsub G gajw gcall goprot grant gt gtu I ica icl in initvlcb insertqueue insphdr intdis intenb irdsq J j n L ladd lb lbx ldc n ldchstatus ldcnt Idconf lddevid ldflags ldiff ldiv ldl n ldlp n Idmemstartval Idnl n ldnlp n ldpi ldpri ldprodid ldresptr ldshadow Idth ldtimer lend lmul ls lshl lshr lsub lsum lsx M mint mkrc move move2dall move2dinit move2dnonzero move2dzero mul N nop norm not O or out outbyte outword P pop prod R readbfr readhdr rem resetch restart ret rev runp S sb selth setchmode sethdr settimeslice shl shr signal ss ssub startp stconf stflags stl n stmove2dinit stnl n stopch stopp stresptr stshadow sttimer sub sum swapbfr swapqueue swaptimer syscall T talt taltwt testpranal timeslice tin tret U unmkrc V vin vout W wait wcnt writehdr wsub wsubdb X xbword xdble xor xsword xword B T9000 instruction set sorted by op-code B.1 Primary functions B.2 Secondary functions B.2.1 Instructions encoded without using prefix B.2.2 Instructions encoded using prefix B.2.3 Instructions encoded using negative prefix Instruction index Index